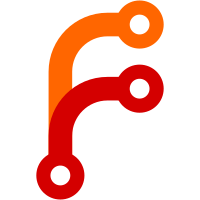
fix repeat added simple keyboard control add preloading tracks add plain view ("api") fix memory leak, add streaming responsive viewport Mobile web capable
68 lines
2.3 KiB
C#
68 lines
2.3 KiB
C#
using System;
|
|
using System.Collections.Generic;
|
|
using System.IO;
|
|
using System.Linq;
|
|
using System.Text.RegularExpressions;
|
|
using System.Web;
|
|
using Microsoft.AspNetCore.Mvc.RazorPages;
|
|
|
|
|
|
namespace webmusic.Pages
|
|
{
|
|
public class IndexModel : PageModel
|
|
{
|
|
public static string root = "music";
|
|
public string path = "";
|
|
public string displaypath = "";
|
|
public string path_oneup = "";
|
|
public string fullpath = "";
|
|
public List<string> dirs = new List<string>();
|
|
public List<string> files = new List<string>();
|
|
public void OnGet()
|
|
{
|
|
if (Request.QueryString.HasValue)
|
|
path = HttpUtility.UrlDecode(Request.QueryString.Value.Remove(0,1));
|
|
if (path.Contains(".."))
|
|
{
|
|
Response.Redirect("/Error");
|
|
return;
|
|
}
|
|
path_oneup = Regex.Match(path, @".*(?=\/)").Value;
|
|
fullpath = root + path;
|
|
displaypath = string.IsNullOrWhiteSpace(path) ? "/" : path;
|
|
dirs = Directory.GetDirectories(fullpath).Select(Path.GetFileName).ToList();
|
|
dirs.Sort();
|
|
files = Directory.GetFiles(fullpath).Select(Path.GetFileName).ToList();
|
|
files.RemoveAll(p => p.EndsWith(".m3u"));
|
|
files.Sort(new NumericComparer());
|
|
}
|
|
|
|
public string Encode(string str)
|
|
{
|
|
return str.Replace("\"", "%22").Replace("'", "%27")
|
|
.Replace("?", "%3F").Replace("&", "%26")
|
|
.Replace(" ", "%20");
|
|
}
|
|
|
|
public class NumericComparer : IComparer<string>
|
|
{
|
|
public int Compare(string x, string y)
|
|
{
|
|
var regex = new Regex(@"^\d+");
|
|
|
|
// run the regex on both strings
|
|
var xRegexResult = regex.Match(x);
|
|
var yRegexResult = regex.Match(y);
|
|
|
|
// check if they are both numbers
|
|
if (xRegexResult.Success && yRegexResult.Success)
|
|
{
|
|
return int.Parse(xRegexResult.Value).CompareTo(int.Parse(yRegexResult.Value));
|
|
}
|
|
|
|
// otherwise return as string comparison
|
|
return string.Compare(x, y, StringComparison.Ordinal);
|
|
}
|
|
}
|
|
}
|
|
} |